Securing GraphQL APIs Against Injection Attacks: A Comprehensive Guide
Master the art of securing GraphQL APIs with our comprehensive guide. This guide will explore the potential vulnerabilities and robust defense strategies to protect against injection attacks. Secure your API endpoints with confidence!
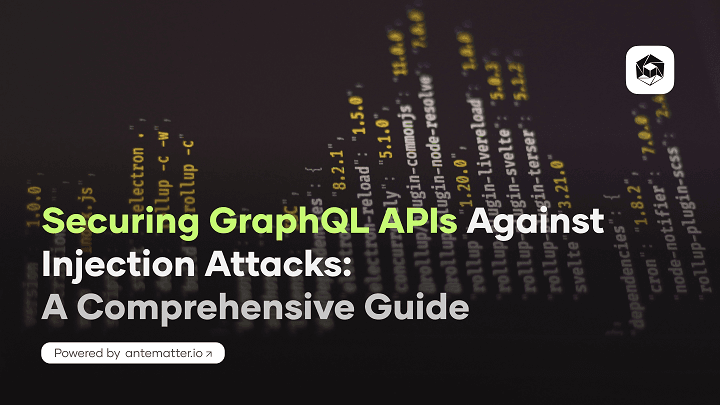
In web development, understanding backend architectures like REST, GraphQL, and gRPC is key for building efficient and secure web applications. REST is known for its simplicity and HTTP method use, serving as a longstanding standard for web APIs.
However, the complexity of modern data interactions has pushed developers towards GraphQL and gRPC. GraphQL, with its single endpoint and data retrieval flexibility, offers a significant improvement in managing data efficiently, allowing clients to request exactly what they need, which can enhance performance and reduce bandwidth. However, this flexibility also introduces new security risks, particularly if the complex querying capabilities are not properly protected.
API security is paramount since APIs are the main gateway for external access to an application's data and functions, making them a prime target for security threats, including injection attacks. Each backend architecture brings its security challenges; for example, GraphQL's complex queries could lead to vulnerabilities without adequate protection.
Developers must thoroughly understand their chosen architecture, be vigilant of potential vulnerabilities, and implement a strong security framework to protect their web applications against various threats. This post focuses on GraphQL, discussing its benefits over REST, the types of attacks it may face, and offering security strategies to strengthen your application. Our goal is to equip you with the knowledge and tools to confidently secure your GraphQL application.
Understanding GraphQL
Introduction to GraphQL
GraphQL is a powerful query language designed for APIs, enabling clients to request precisely the data they need. This innovative approach not only streamlines data retrieval processes but also addresses common limitations faced by REST, such as over-fetching or under-fetching of data.
Working Principles
At its core, GraphQL operates through a single endpoint that interprets and fulfills queries, allowing for dynamic data fetching. Clients can specify exact fields and relationships, leading to more efficient data exchanges. Here’s an example illustrating a GraphQL query in action fetching the names of movies and their directors from a movie API.
This specificity ensures that clients receive only the necessary data, like the movies and their director's name, without extraneous information.
Key Features Distinguishing GraphQL from REST:
- Single Endpoint for Queries: Unlike REST, which uses multiple endpoints, GraphQL utilizes a single endpoint for various data requests, simplifying the API structure.
- Specific Data Fetching: Clients have the liberty to request exactly what they need, reducing unnecessary data transfer.
- Efficient Data Retrieval: By allowing clients to specify fields, GraphQL minimizes over-fetching and under-fetching issues prevalent in REST APIs.
Attack Scenarios with GraphQL
Despite its benefits, GraphQL is still vulnerable to security risks and threats. It introduces new vulnerabilities for exploitation, but it's also important to strengthen defenses against common web application threats that can impact GraphQL operations.
In our exploration, we will delve into various attack scenarios specific to GraphQL and discuss strategies to mitigate these risks.
By understanding these vulnerabilities and implementing robust defenses, we can ensure the security and integrity of applications powered by GraphQL.
Introspection Attack
The GraphQL schema defines the structure of an API, outlining data types, queries, and mutations. It's accessible through introspection, a feature enabled by default in most GraphQL APIs, which allows anyone to query the API's schema. While introspection is crucial for understanding and using a GraphQL API, it can also expose detailed schema information to potential attackers.
To perform introspection, a simple HTTP request with a specific introspection query is sent, revealing the API's full schema, including all queries, data types, and mutations. This powerful feature aids in API exploration but requires careful management to prevent unwanted information disclosure.
Here’s an example of an introspection
The result of such a query reveals the following information.
A full graphQL Introspection query can be found here.
GraphiQL
GraphiQL is an IDE-like interface for building and testing GraphQL queries, featuring auto-completion and schema documentation. Commonly enabled by default, it can be found at various endpoints like /graphiql, /playground, or /console. While useful for developers, it also poses a security risk by potentially aiding attackers in accessing your API schema for information gathering.
Excessive Errors/Fields Suggestions
GraphQL error messages often provide detailed information, which can inadvertently guide attackers in crafting valid queries or attacks. For example, error descriptions may reveal available fields for specific types, like "Directors," potentially aiding unauthorized access.
Suppose we request something like this from a query
The response from graphQL is returned as such, revealing information from schema.
Denial of Service
GraphQL APIs may face Denial of Service (DoS) attacks, with attackers flooding the server with requests that can overload the application, exploiting GraphQL's operational model. We will explore the following types of DoS attacks.
Batching Attacks
GraphQL allows sending multiple queries in one HTTP request through query batching. This can lead to DoS attacks as a single request can trigger numerous backend queries, potentially overloading the server by exhausting resources like CPU or memory.
For instance, an attacker might use array-based batching to call a resource-intensive function multiple times in one request:
This method can circumvent rate limits, which typically count HTTP requests, by consolidating many calls into a single network request.
Alias Overloading
Disabling batching doesn't fully protect a server from being overwhelmed, as attackers can exploit aliases to simulate batching. Aliases allow renaming fields in queries, enabling multiple instances of the same query with different parameters or names in a single request. This technique can overload the server by executing numerous queries at once.
Example using GraphQL aliases to overwhelm the server:
Circular Queries
In GraphQL, circular or cyclic queries occur when types reference each other, potentially creating exponentially complex queries. Unchecked, these can heavily burden or crash the server, posing a risk of DoS attacks. Circular queries involve fields that reference back to themselves, consuming significant resources.
The above circular query example fetches a user, their friends, and their friends' friends recursively, potentially creating an infinite loop. This can overload the server by endlessly querying interconnected data without a set limit
Injection
Injection attacks in GraphQL involve attackers exploiting security flaws to inject and run unauthorized commands or code within an API. These attacks target weaknesses in how a GraphQL server processes user-provided input.
Now we will look at the following injection attacks that are possible in graphQL.
SQL Injection
GraphQL SQL injection attacks exploit inadequate input sanitization in GraphQL APIs, allowing attackers to run harmful SQL queries on the backend database. By injecting malicious SQL into GraphQL queries, attackers can bypass security measures, execute unauthorized queries, and manipulate sensitive data.
Example:
In this example, the attacker injects "1 OR '1'='1" into the search parameter. If the input is directly used in an SQL query without proper sanitization, it effectively alters the query logic to return all users, bypassing any intended restrictions.
NoSQL injection
NoSQL injection attacks exploit systems using NoSQL databases, similar to SQL injection in principle. Attackers manipulate GraphQL queries that accept JSON inputs for searching data, like usernames or email addresses, by injecting database-specific commands.
In this example, an attacker changes the search parameter to {"email": {"$exists": true}}
, targeting a MongoDB or similar NoSQL database. This query could potentially return all user details in the system, exploiting the unsanitized JSON input to bypass security controls and indiscriminately access or manipulate sensitive data.
Cross-Site Scripting
XSS attacks happen when malicious scripts are embedded into reputable websites. Consider an attacker inserting a script into a comment field:
This leads to the script executing in a user's browser upon viewing the comment, demonstrating how XSS vulnerabilities can be exploited through user inputs like comments.
OS Command Injection
OS command injection is a vulnerability enabling attackers to run any commands on a server hosting an application. This issue arises when an application sends unsanitized user input directly to the OS for execution.
Within GraphQL, this vulnerability might manifest through mutations or queries that incorporate user input into system commands without proper validation or sanitization. Suppose a GraphQL API enables users to request file information by passing a filename to a system command.
An attacker injects a harmful command (; rm -rf /*) alongside a benign filename, exploiting the lack of input sanitization and resulting in removing the directory of the server.
Mitigating Security Flaws in GraphQL
As we conclude our exploration of GraphQL APIs and the attack scenarios possible in it, it's clear that vigilance and a proactive security stance are paramount. The journey toward a secure GraphQL application encompasses a spectrum of practices, from disabling introspection in production environments to advanced input validation and access control measures.
Key Takeaways for Enhancing GraphQL Security:
- Limit Exposure: Disable introspection and development tools like GraphiQL in live environments to minimize information leakage.
- Error Management: Craft error messages that are informative for development purposes yet opaque to external users, using middleware to filter out sensitive information.
- Query Control: Regulate query batching and alias usage to prevent abuse that could lead to performance degradation or server exploitation.
- Visualize and Limit: Employ schema visualization tools to identify and mitigate potential vulnerabilities, and implement query depth limiting to avoid overly complex, resource-intensive queries.
- Rigorous Input Handling: Prioritize the validation and sanitization of user inputs to defend against injection attacks, ensuring that only sanitized data interacts with your database and server.
- Access Control: Strengthen your API with strict server-side validation, authentication, and authorization practices, ensuring that only authorized entities can access your data.
Final Thoughts:
The commitment to securing a GraphQL API is not a one-time effort but a continuous process that evolves with emerging threats and new security insights. Leveraging tools, adopting best practices, and maintaining an awareness of the security landscape are crucial steps in building and sustaining robust defenses for your GraphQL applications.